Our Goal
Whenever a Github repository is created there are a number of tasks that are required to complete. If forgotten, the repository is at risk of error. Automating these tasks would save time and improve consistency between repos, increasing reliability and decreasing the chance for errors. This line of thought has allowed us to explore further opportunities for automation in an effort to save time and reduce error.
The GitHub API
Scouting out GitHub’s API and finding out what type of settings we can automate was essential. GitHub’s extensive API and perfect timing for the full release of a branch protection API, I found out that they had support calls for everything we need. This included; editing the protection on a branch, creating labels and editing base repo settings such as the type of merges that are permitted. While looking at that I took a look at GitHub’s web hooks and saw that they can send us specific events when they occur.
During this period, I also observed our current repos and found inconsistencies such as unprotected master branches and voluntary reviews of code before merge into a master. Making these reviews voluntary instead of compulsory means the codebase might have inconsistencies when merged with the master.
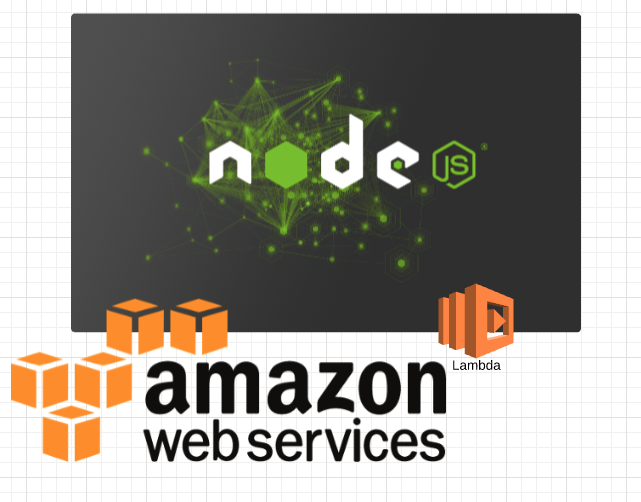
The framework
After the research, I decided what platform we were going to use. I figured that we’d need a way for GitHub to notify us, meaning we needed an API gateway. For this reason, we have decided to create an API gateway using node.js with express.js to get an easy and fast gateway up and running. After setting that up and configuring the application to our liking, we used apex/up as the serverless framework to deploy the application straight to the hosting with AWS Lambda.
Setting up the node application is as simple as installing express.js and then creating a server off of that. Here’s some code to get you started